How to Bind Data in Maui C#: A Beginner's Guide with Examples
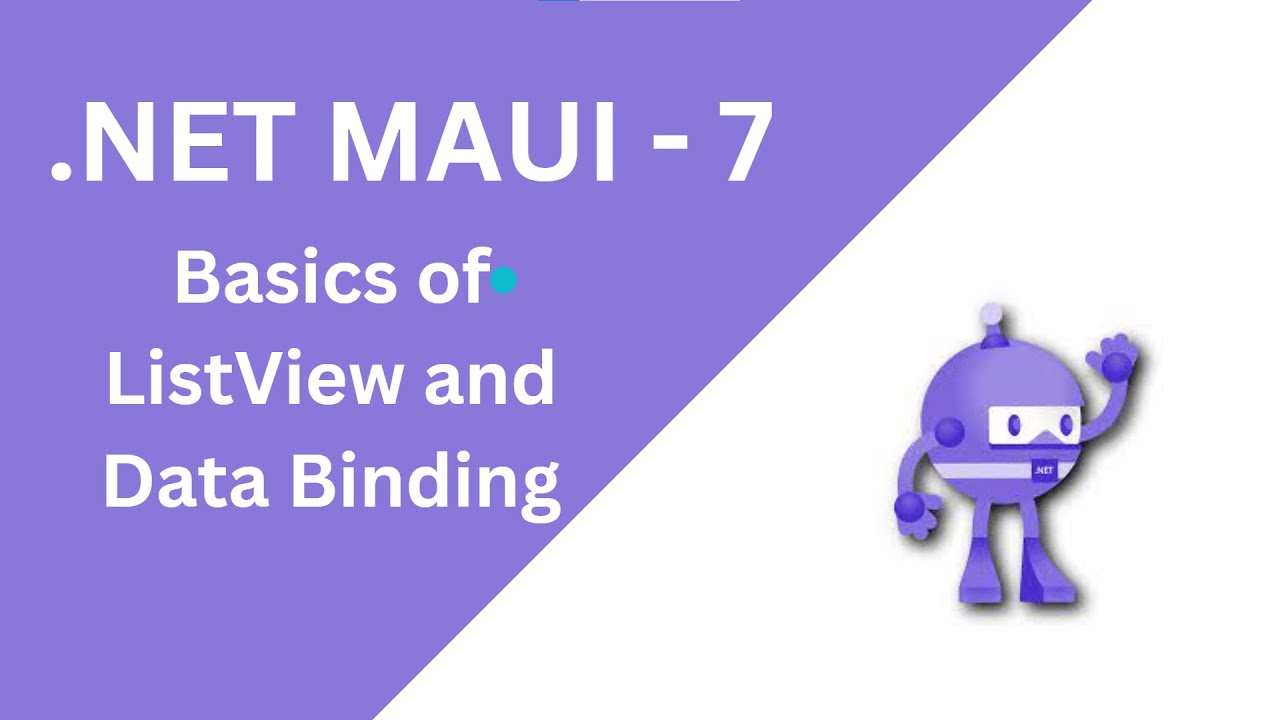 |
How to Bind Data in Maui C# |
Have you ever had to manually update the contents of a UI element in your Maui C# application every time the data it displays changes? It can be time-consuming and error-prone. Fortunately, there's a better way: data binding.
Data binding is a technique that allows you to link the data in your application's data model with the UI elements that display that data. When the data changes, the UI elements automatically update to reflect the new data, without any additional code from you.
In this beginner's guide, we'll walk you through how to use data binding in Maui C# step-by-step, with plenty of examples to help you understand each concept.
Steps to Bind Data in Maui c# :
Step 1: Create a Data Model
The first step in using data binding is to create a data model. A data model is a class that defines the properties and methods that your application will use to interact with the data. For example, if your application displays a list of customers, your data model might include properties such as Name, Address, and Phone Number.
To create a data model, you'll need to define a class that includes the properties you want to use. Here's an example:
public class Customer
{
public string Name { get; set; }
public string Address { get; set; }
public string PhoneNumber { get; set; }
}
Step 2: Create a View Model
The next step is to create a view model. A view model is a class that represents the data that your UI elements will display. It's responsible for retrieving the data from the data model and exposing it to the UI elements.
To create a view model, you'll need to define a class that includes a property for each piece of data that your UI elements will display. Here's an example:
public class CustomerViewModel
{
private Customer _customer;
public string Name
{
get { return _customer.Name; }
set
{
if (_customer.Name != value)
{
_customer.Name = value;
OnPropertyChanged();
}
}
}
public string Address
{
get { return _customer.Address; }
set
{
if (_customer.Address != value)
{
_customer.Address = value;
OnPropertyChanged();
}
}
}
public string PhoneNumber
{
get { return _customer.PhoneNumber; }
set
{
if (_customer.PhoneNumber != value)
{
_customer.PhoneNumber = value;
OnPropertyChanged();
}
}
}
public CustomerViewModel(Customer customer)
{
_customer = customer;
}
public event PropertyChangedEventHandler PropertyChanged;
protected void OnPropertyChanged([CallerMemberName] string propertyName = "")
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
Note that in this example, we're using the INotifyPropertyChanged interface to notify the UI elements when the data changes. This interface is required for data binding to work properly.
Step 3: Link the View Model to the UI Elements
Once you've created your view model, you'll need to link it to the UI elements that will display the data. You can do this by setting the DataContext property of the UI element to an instance of the view model.
For example, if you have a TextBlock that displays the customer's name, you can set its DataContext property like this:
var customer = new Customer { Name = "John Doe", Address = "123 Main St", PhoneNumber = "555-555-1212" };
var viewModel = new CustomerViewModel(customer);
myTextBlock.DataContext = viewModel;
Step 4: Bind the UI Elements to the View Model Properties
Now that you've linked the view model to the UI elements, you can bind the UI elements to the properties of the view model. To do this, you'll use data binding expressions.
A data binding expression is a string that specifies how to bind the UI element to the view model property. The syntax for a data binding expression is {Binding PropertyName}.
For example, to bind the Text property of a TextBlock to the Name property of the view model, you can set the Text property like this:
<TextBlock Text="{Binding Name}" />
When the data changes, the TextBlock will automatically update to reflect the new value of the Name property.
Step 5: Implement Two-Way Data Binding
In some cases, you'll want to allow the user to edit the data displayed in the UI elements and have those changes automatically update the data model. This is called two-way data binding.
To implement two-way data binding, you'll need to add the Mode=TwoWay parameter to the data binding expression. For example:
<TextBox Text="{Binding Name, Mode=TwoWay}" />
Now when the user edits the text in the TextBox, the Name property of the view model will be automatically updated to reflect the new value.
Step 6: Use Converters to Format Data
Sometimes you'll want to format the data displayed in the UI elements in a specific way. For example, you might want to display a date in a specific format or convert a numeric value to a string.
You can use data-binding converters to do this. A data binding converter is a class that implements the IValueConverter interface. It takes a value as input and returns a formatted version of that value.
For example, here's a converter that converts a DateTime value to a string in the format "MM/dd/yyyy":
public class DateTimeConverter : IValueConverter
{
public object Convert(object value, Type targetType, object parameter, CultureInfo culture)
{
if (value is DateTime dateTimeValue)
{
return dateTimeValue.ToString("MM/dd/yyyy");
}
return value;
}
public object ConvertBack(object value, Type targetType, object parameter, CultureInfo culture)
{
throw new NotImplementedException();
}
}
To use this converter, you'll need to create an instance of it and add it to the Resources collection of the UI element. Then you can use it in a data binding expression like this:
<TextBlock Text="{Binding Date, Converter={StaticResource DateTimeConverter}}" />
Step 7: Use Observable Collections for Dynamic Data
If you're displaying a list of data in your UI, you'll need to use an ObservableCollection instead of a regular list. An ObservableCollection is a special type of list that automatically updates the UI when items are added or removed from the list.
To use an ObservableCollection, you'll need to create a new class that inherits from it. Here's an example:
public class CustomerList : ObservableCollection<Customer>
{
}
Then you can use this class to store your list of customers, like this:
var customerList = new CustomerList();
customerList.Add(new Customer { Name = "John Doe", Address = "123 Main St", PhoneNumber = "555-555-1212" });
And you can bind a ListView to this collection like this:
<ListView ItemsSource="{Binding Customers}" />
Conclusion
In this beginner's guide, we've covered the basics of data binding in Maui C#. We've shown you how to create a view model, bind UI elements to view model properties, implement two-way data binding, use converters to format data, and use observable collections for dynamic data.
By following these steps, you can create a data-driven UI that automatically updates to reflect changes in your data model. Whether you're building a simple form or a complex application, data binding is an essential tool in your toolbox.